Mastering Python: 10 Advanced Tips and Tricks for Senior Developers
Python is a powerful programming language that offers simplicity and versatility, making it a favorite among developers. As a senior developer, you have likely mastered the basics and are ready to dive deeper into advanced techniques that can refine your coding skills and enhance your productivity. This guide provides ten advanced tips and tricks that will help you sharpen your expertise and fully leverage the potential of Python.
1. Embrace Python's Iterators and Generators
Iterators and generators are powerful tools in Python that improve memory efficiency, especially when dealing with large datasets. While an iterator is an object that implements the __iter__()
method, allowing you to traverse through a container, a generator creates an iterator without storing all elements in memory at once.
For instance, you can use a generator expression to efficiently generate a series of numbers:
def natural_numbers(max):
num = 0
while num < max:
yield num
num += 1
Generators are useful for creating infinite sequences and handling large data without running out of memory.
2. Master List Comprehensions and Dictionary Comprehensions
List comprehensions offer a concise way to create lists and are more efficient than traditional loops. They are not limited to lists; similar syntax can be applied to dictionaries, sets, and generators.
Here is a basic example of a list comprehension:
numbers = [i**2 for i in range(10)]
And for dictionary comprehensions:
words = ['Python', 'Java', 'C++']
word_count = {word: len(word) for word in words}
Understanding comprehensions allows for cleaner and faster code writing.
3. Optimize Code with Built-in Functions
Python's built-in functions like map()
, filter()
, and reduce()
are great for processing iterables efficiently. These functions can leverage performance by executing operations faster in C-based Python internals than a manual loop in Python.
For example, map()
can apply a function to all elements in a list:
results = map(lambda x: x ** 2, range(10))
These functions promote functional programming patterns, often leading to more elegant solutions.
4. Deep Dive into Async Programming
Leverage asynchronous programming to handle tasks that involve waiting for external resources, such as web requests or file I/O, more efficiently. Python's asyncio
library provides the core infrastructure for writing async code.
Understanding how to define an async function:
import asyncio
async def fetch_data():
await asyncio.sleep(1)
return 'Data Retrieved'
This approach boosts performance by allowing your program to continue executing other tasks while waiting.
5. Implement Decorators for Reusability
Decorators allow you to modify the behavior of functions or methods. They are particularly beneficial for adding logging, authentication, or caching without altering the function code.
Here's an example of a simple decorator:
def log_decorator(func):
def wrapper(*args, **kwargs):
print(f'Calling {func.__name__}')
return func(*args, **kwargs)
return wrapper
Decorators bring about higher reusability and clean abstract code layers.
6. Leverage Python's Robust Data Libraries
For handling complex data analysis, take advantage of Python's rich ecosystem of libraries like Pandas
, NumPy
, and Matplotlib
. These libraries are optimized for performance and feature a plethora of tools for data manipulation and visualization.
The use of Pandas
and NumPy
can lead to significant time savings when working with large datasets:
import pandas as pd
import numpy as np
data = pd.DataFrame(np.random.rand(1000, 5), columns=list('ABCDE'))
Integrating these tools can lead to more powerful data insights.
7. Strengthen Code Quality with Testing and Linting
Maintaining high code quality is crucial, and testing is a fundamental part of the process. Utilize unittest
or pytest
for automated testing frameworks to validate your code:
import unittest
class TestMath(unittest.TestCase):
def test_addition(self):
self.assertEqual((1 + 2), 3)
Code linters such as flake8
or pylint
can further enforce coding standards.
Testing ensures your code remains reliable and maintainable.
8. Modularize Code with Packages
Code packaged into modules enhances reusability and organization. Python projects can be structured into packages, grouping similar functionalities together and promoting code organization:
Structure example:
my_project/
__init__.py
module_one.py
module_two.py
This approach fosters readability and seamless collaboration among team members.
9. Adopt Type Hints for Clarity
Type hints improve the clarity and debugging efficiency by specifying expected data types of function arguments and return values, integrated into Python via PEP 484
. For example:
def greeting(name: str) -> str:
return f'Hello, {name}'
Though not mandatory due to Python's dynamic typing nature, adding type hints can enhance readability and assist IDEs in providing better support.
10. Secure Your Code
Security has never been more crucial. To secure your Python applications, follow best practices such as restricting dangerous modules, validating inputs, and managing dependencies with tools like pip-audit
.
Implementing security measures like SSL/TLS
can help secure data in transit and employing a vigilant approach to dependency management avoids vulnerabilities.
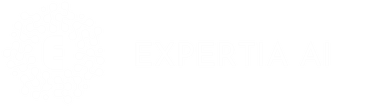
Made with from India for the World
Bangalore 560101
© 2025 Expertia AI. Copyright and rights reserved
© 2025 Expertia AI. Copyright and rights reserved